p5 has many built-in functions to draw different shapes. In this example, we are drawing a rectangle.
The rect function takes four parameters: x, y, width and height. The x and y values refer to location on the canvas and the width and height refer to size.
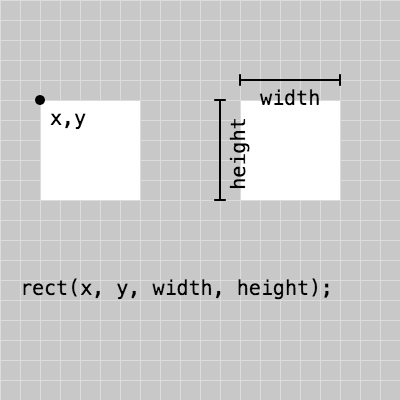
Recreate the following example and play with the parameter values.
Example
To help you place shapes where you want them on the canvas, you can add the following line to your code:
text(mouseX + ", " + mouseY, 20, 20)
Take a look at the example below. You can move your mouse to where you would like your shape to be, and write down the coordinates to use them as parameters in your function call.
You also use this blank grid template to help you position your shapes.